Foreign key between source table and target table.
const relation = product.M2ORelationRelations[0]; // RELATION: line_item >---- product
const foreignKey = relation.foreignKey; // CONSTRAINT: ^-- product_has_carts
const FKColumn = relation.foreignKey.columns[0]; // COLUMN: product_id (from line_item table)
Whether the relation targets to many. Since, many to one relations targets single, this is true
.
Type of the relation, which is m2o
for M2ORelation.
For TypeScript it is enum of RelationType.M2O
.
Informational text representation of the relation.
const info = relation.info; // [public.cart] ⭃―― cart_contact ―――[public.contact]
Suggested name for relation.
Source table's adjective extracted from foreign key name.
Source table alias
Source table name.
Source table's adjective extracted from foreign key name.
Target table alias
Target table name
Returns name for the relation using given naming function.
are custom functions or name of the module that exports relation name functions to generate names with. pg-structure
provides some builtin modules (short
, optimal
and descriptive
), but you can use your own.
name for the relation using naming function.
Returns source table alias after replacing given tables' names from it.
is type or types of tables to exclude names of.
source table alias after given tables' names replaced.
Returns source table name after replacing given tables' names from it.
is type or types of tables to exclude names of.
source table name after given tables' names replaced.
Returns target table alias after replacing given tables' names from it.
is type or types of tables to exclude names of.
target table alias after given tables' names replaced.
Returns target table name after replacing given tables' names from it.
is type or types of tables to exclude names of.
target table name after given tables' names replaced.
Generated using TypeDoc
Class which represent many to one relationship which resembles
belongsTo
relation in ORMs (Object Relational Mappers). Provides attributes and methods for details of the relationship.Actually there is no many to one relation in database engine. It is basically one to many relation in reverse direction.
Below is a database schema as an example: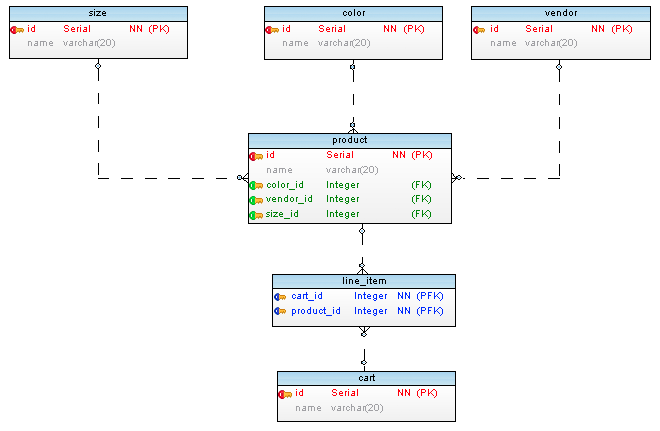
Some definitions used in descriptions for M2ORelation.
Example
```typescript // Example tables have single primary key and examples first relation. So zero index ([0]) is used. Use all array elements if necessary. // line_item >---- product // (source) (target)const relation = line_item.m2oRelations[0]; // RELATION: line_item >---- product const foreignKey = relation.foreignKey; // CONSTRAINT: ^-- product_has_carts const sourceTable = relation.sourceTable; // TABLE: line_item const targetTable = relation.targetTable; // TABLE: product const FKColumn = relation.foreignKey.columns[0]; // COLUMN: product_id (from line_item table) const PKColumn = relation.targetTable.primaryKeys[0]; // COLUMN: id (from product table) ```