All columns of the entity as an {@link IndexableArray indexable array} ordered by same order they are defined in database entity.
const isAvailable = table.columns.has('id');
const columnNames = table.columns.map(column => column.name);
const column = table.columns.get('user_id');
const name = column.name;
table.columns.forEach(column => console.log(column.name));
Comment of the database object defined in database including {@link DbObject#commentData comment data}.
All constraints in the table as an [[IndexableArray]] ordered by name.
All foreign keys which are referring to this table as an [[IndexableArray]].
All indexes in the table as an [[IndexableArray]], ordered by name.
Name of the database object.
Object identifier for the Entity
Schema of the object.
All triggers in the table as an [[IndexableArray]] ordered by name.
Tables which this table has many to many relationship, ordered by table name
Please note that same table will appear more than once if there are more than one relationship between tables or same named tables from
different schemas (i.e. public.account
may have relations with public.contact
and vip.contact
).
// Cart (id) has many products (id) through line_item join table.
cartTable.belongsToManyTables.forEach(table => console.log(table.name));
cartTable.belongsToManyTables.getAll("contact"); // All related tables named contact.
Tables which this table has many to many relationship joined by primary keys only in join table, ordered by table name
Please note that same table will appear more than once if there are more than one relationship between tables or same named tables from
different schemas (i.e. public.account
may have relations with public.contact
and vip.contact
).
// Cart (id) has many products (id) through line_item join table.
cartTable.belongsToManyTablesPk.forEach(table => console.log(table.name));
cartTable.belongsToManyTablesPk.getAll("contact"); // All related tables named contact.
Tables, which this table has belongs to relationship (a.k.a. many to one) which is reverse direction of
one to many relationship (a.k.a one to many), ordered by table name.
Please note that same table will appear more than once if there are more than one relationship between tables or same named tables from
different schemas (i.e. public.account
may have relations with public.contact
and vip.contact
).
productTable.belongsToTables.forEach(table => console.log(table.name));
productTable.belongsToTables.getAll("contact"); // All related tables named contact.
All check constraints in the table as an [[IndexableArray]] ordered by name.
Data which is extracted from database object's comment. Data is extracted from text between special case-insensitive tag
(default: [pg-structure][/pg-structure]
) and converted to JavaScript object using JSON5.
Token name can be specified by using commentDataToken
arguments.
For details of JSON5, see it's web site: https://json5.org.
// "Account details. [pg-structure]{ extraData: 2 }[/pg-structure] Also used for logging."
table.comment; // "Account details. [pg-structure]{ extraData: 2 }[/pg-structure] Also used for logging."
table.commentWithoutData; // "Account details. Also used for logging."
table.commentData; // { extraData: 2 }
table.commentData.extraData; // 2
Description or comment of the database object defined in database. If comment contains {@link DbObject#commentData comment data}, it is removed.
// "Account details. [pg-structure]{ extraData: 2 }[/pg-structure] Also used for logging."
table.commentWithoutData; // "Account details. Also used for logging."
All exclusion constraints in the table as an [[IndexableArray]] ordered by name.
All foreign keys in the table as an [[IndexableArray]] ordered by name.
Full name of the database object including database name.
Tables, which this table has one to many relationship, ordered by table name.
Please note that same table will appear more than once if there are more than one relationship between tables or same named tables from
different schemas (i.e. public.account
may have relations with public.contact
and vip.contact
).
vendorTable.hasManyTables.forEach(table => console.log(table.name));
vendorTable.hasManyTables.getAll("contact"); // All related tables named contact.
Array of many to many relationships of the table. Many to many relationships resembles
has many through
and belongs to many
relations in ORMs. It has some useful methods and information for generating ORM classes.
Array of many to many relationships of the table. Different from m2mRelations,
this only includes relations joined by Primary Foreign Keys
in join table. Primary Foreign Key
means
foreign key of join table which are also primary key of join table at the same time.
M2MRelation resembles has many through
and belongs to many
relations in ORMs.
It has some useful methods and information for generating ORM classes.
Array of many to one relationships of the table. M2ORelation resembles
belongs to
relations in ORMs. It has some useful methods and information for generating ORM classes.
Letter casing (i.e snakeCase
or camelCase
) of the database object name.
const name = entity.name; // ProductDetail
const caseType = entity.nameCaseType; // camelCase
const otherEntity = otherEntity.name; // member_protocol
const otherCaseType = otherEntity.nameCaseType; // snakeCase
Array of one to many relationships of the table. O2MRelation resembles
has many
relations in ORMs. It has some useful methods and information for generating ORM classes.
Primary key of this table.
table.primaryKey.columns.forEach(column => console.log(column.name));
List of all relationships of the table. They are sort by type (O2MRelation, M2ORelation, M2MRelation).
Separator used in database object name. Empty string for came case and underscore for (_) snake case.
All unique constraints in the table as an [[IndexableArray]] ordered by name.
Returns foreign keys from given table to this table.
foreign keys from given table to this table.
Returns foreign keys from this table to target
table.
foreign keys from this table to target table.
Returns through constraints.
is whether to include only PK column constraints.
through constraints and their details.
Generated using TypeDoc
Class which represent a table. Provides attributes and methods for details of the table. Tables may have relationships with other tables.
Below is a database schema which is used in code examples.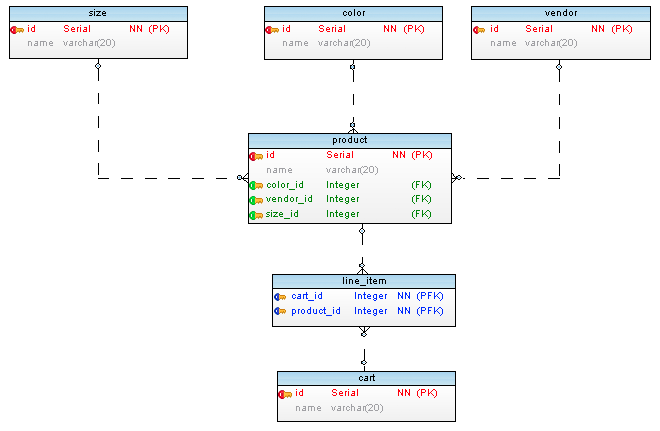